Physical Computing
Physical computing is the process of using hardware and software to build devices inorder to interact with real world.
it involves :
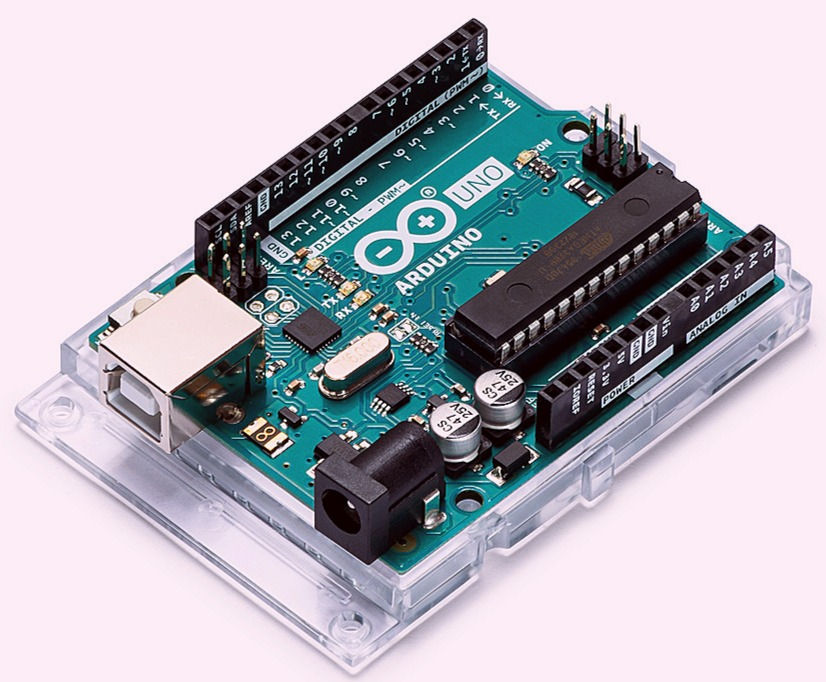
A microcontroller (Arduino Uno)
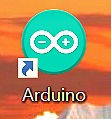
Associated software (Arduino platform) to
implement your program.
Activities using www.tinkercad.com
1. Blink Example
LEDs are polarized, they only work if they are the correct way round. Both the external RED LED and the built-in LED are blinking.
Blink Challenges
1. Make the LED blink at different rates
Code:
// C++ code
//
void setup()
{
pinMode(LED_BUILTIN, OUTPUT);
}
void loop()
{
digitalWrite(LED_BUILTIN, HIGH);
delay(1500); // Wait for 1000 millisecond(s)
digitalWrite(LED_BUILTIN, LOW);
delay(500); // Wait for 1000 millisecond(s)
}
2. Use two LEDs and make them flash alternately
Code:
#define ledRed 12
#define ledGreen 13
void setup() {
pinMode(ledRed, OUTPUT);
pinMode(ledGreen, OUTPUT);
}
void loop() {
digitalWrite(ledRed, 1);
digitalWrite(ledGreen, 0);
delay(1000);
digitalWrite(ledRed, 0);
digitalWrite(ledGreen, 1);
delay(1000);
}
2. Simple Button
Can you modify the code so the LED will turn ON when the button is pressed?
Comments